Babylon JS Day 11
I’m kicking off Week 3 of A Month of Babylon JS by diving into a week-long project to present some data as interactive cards in 3D. My Friday Project this week will involve building some VR interaction for these cards. For now, I’m going to focus on the data and the layout of the cards.
Getting the data
In the interest of saving time, I decided to use the blog posts in this series as the data source for the cards. Rather than spend any time on an API to build this data, I used the XML in my RSS feed. Since my site is built on WordPress, the RSS feed has query parameters built right in. All the posts in this series are tagged with a value of babylon-month
so the url to get the data is:
https://radicalappdev.com/feed/?tag=babylon-month
I’m not spending any time on the backend of this project, so I’ll just serve the data as a JSON file. I converted it from the RSS XML to JSON with this site. I know… Lazy Joe. The data is hosted on my WebXR Sandbox site here.
Loading the data
Something that caught my attention last week was a reference to a method called addTextFileTask
on the Asset Manager. I can use this to load the JSON file, then parse the text as JSON, and pass it to a function that will iterate over it to create cards in the scene.
var assetsManager = new BABYLON.AssetsManager(scene);
const dataPath = "https://webxr.radicalappdev.com/assets/data/babylon-month.json";
assetsManager.addTextFileTask("babylon-month", dataPath).onSuccess = function (task) {
const parsed = JSON.parse(task.text);
createCards(scene, parsed.items); // this function will append 3d card objects to the scene
};
assetsManager.load();
3D GUI in Babylon JS
I didn’t have much time left to work on the cards today, but I got a head start on tomorrow by checking out the 3D GUI features of Babylon JS. For now, I just used some sample code from the Plane Panel example. This will create a panel object, then loop over the array of items, creating a HolographicButton
object for each one. Not perfect, but good enough for today.
function createCards(scene, items) {
// Create the 3D UI manager
var anchor = new BABYLON.TransformNode("");
var manager = new BABYLON.GUI.GUI3DManager(scene);
var panel = new BABYLON.GUI.PlanePanel();
panel.margin = 0.2;
manager.addControl(panel);
panel.linkToTransformNode(anchor);
panel.position.z = -1.5;
// Let's add some buttons!
var addButton = function (item) {
var button = new BABYLON.GUI.HolographicButton("orientation");
panel.addControl(button);
button.text = item.title;
};
panel.blockLayout = true;
for (item of items) {
console.log(item);
addButton(item);
}
panel.blockLayout = false;
}
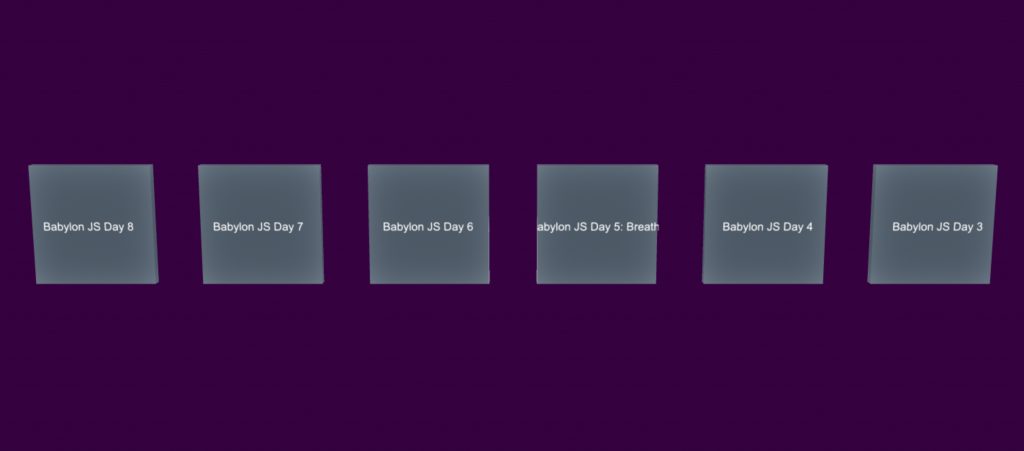
Tomorrow I’ll start working on the 3D card object itself. Then I’ll replace the buttons in the sample code above with the new object.